c6b8a28f10e865c8444e9e8ffb6eee5dbd428192,test/augmenters/test_blur.py,Test_blur_gaussian_,test_other_dtypes_sigma_075,#Test_blur_gaussian_#,190
Before Change
"int8", "int16", "int32",
"float16", "float32", "float64"]
]
for backend, dtypes_to_test in zip(["scipy", "cv2"], dtypes_to_test_list):
// bool
if "bool" in dtypes_to_test:
with self.subTest(backend=backend, dtype="bool"):
image = np.zeros((5, 5), dtype=bool)
image[2, 2] = True
image_aug = iaa.blur_gaussian_(np.copy(image), sigma=0.75, backend=backend)
assert image_aug.dtype.name == "bool"
assert np.all(image_aug == (mask > 0.5))
// uint, int
for dtype in [np.uint8, np.uint16, np.uint32, np.uint64, np.int8, np.int16, np.int32, np.int64]:
dtype = np.dtype(dtype)
if dtype.name in dtypes_to_test:
with self.subTest(backend=backend, dtype=dtype.name):
min_value, center_value, max_value = iadt.get_value_range_of_dtype(dtype)
dynamic_range = max_value - min_value
value = int(center_value + 0.4 * max_value)
image = np.zeros((5, 5), dtype=dtype)
image[2, 2] = value
image_aug = iaa.blur_gaussian_(image, sigma=0.75, backend=backend)
expected = (mask * value).astype(dtype)
diff = np.abs(image_aug.astype(np.int64) - expected.astype(np.int64))
assert image_aug.shape == mask.shape
assert image_aug.dtype.type == dtype
if dtype.itemsize <= 1:
assert np.max(diff) <= 4
else:
assert np.max(diff) <= 0.01 * dynamic_range
// float
values = [5000, 1000**1, 1000**2, 1000**3]
for dtype, value in zip([np.float16, np.float32, np.float64, np.float128], values):
dtype = np.dtype(dtype)
if dtype.name in dtypes_to_test:
with self.subTest(backend=backend, dtype=dtype.name):
image = np.zeros((5, 5), dtype=dtype)
image[2, 2] = value
image_aug = iaa.blur_gaussian_(image, sigma=0.75, backend=backend)
expected = (mask * value).astype(dtype)
diff = np.abs(image_aug.astype(np.float128) - expected.astype(np.float128))
assert image_aug.shape == mask.shape
After Change
"int8", "int16", "int32",
"float16", "float32", "float64"]
]
gen = zip(["scipy", "cv2"], dtypes_to_test_list)
for backend, dtypes_to_test in gen:
// bool
if "bool" in dtypes_to_test:
with self.subTest(backend=backend, dtype="bool"):
image = np.zeros((5, 5), dtype=bool)
image[2, 2] = True
image_aug = iaa.blur_gaussian_(
np.copy(image), sigma=0.75, backend=backend)
assert image_aug.dtype.name == "bool"
assert np.all(image_aug == (mask > 0.5))
// uint, int
uint_dts = [np.uint8, np.uint16, np.uint32, np.uint64]
int_dts = [np.int8, np.int16, np.int32, np.int64]
for dtype in uint_dts + int_dts:
dtype = np.dtype(dtype)
if dtype.name in dtypes_to_test:
with self.subTest(backend=backend, dtype=dtype.name):
min_value, center_value, max_value = \
iadt.get_value_range_of_dtype(dtype)
dynamic_range = max_value - min_value
value = int(center_value + 0.4 * max_value)
image = np.zeros((5, 5), dtype=dtype)
image[2, 2] = value
image_aug = iaa.blur_gaussian_(
image, sigma=0.75, backend=backend)
expected = (mask * value).astype(dtype)
diff = np.abs(image_aug.astype(np.int64)
- expected.astype(np.int64))
assert image_aug.shape == mask.shape
assert image_aug.dtype.type == dtype
if dtype.itemsize <= 1:
assert np.max(diff) <= 4
else:
assert np.max(diff) <= 0.01 * dynamic_range
// float
float_dts = [np.float16, np.float32, np.float64, np.float128]
values = [5000, 1000**1, 1000**2, 1000**3]
for dtype, value in zip(float_dts, values):
dtype = np.dtype(dtype)
if dtype.name in dtypes_to_test:
with self.subTest(backend=backend, dtype=dtype.name):
image = np.zeros((5, 5), dtype=dtype)
image[2, 2] = value
image_aug = iaa.blur_gaussian_(
image, sigma=0.75, backend=backend)
expected = (mask * value).astype(dtype)
diff = np.abs(image_aug.astype(np.float128)
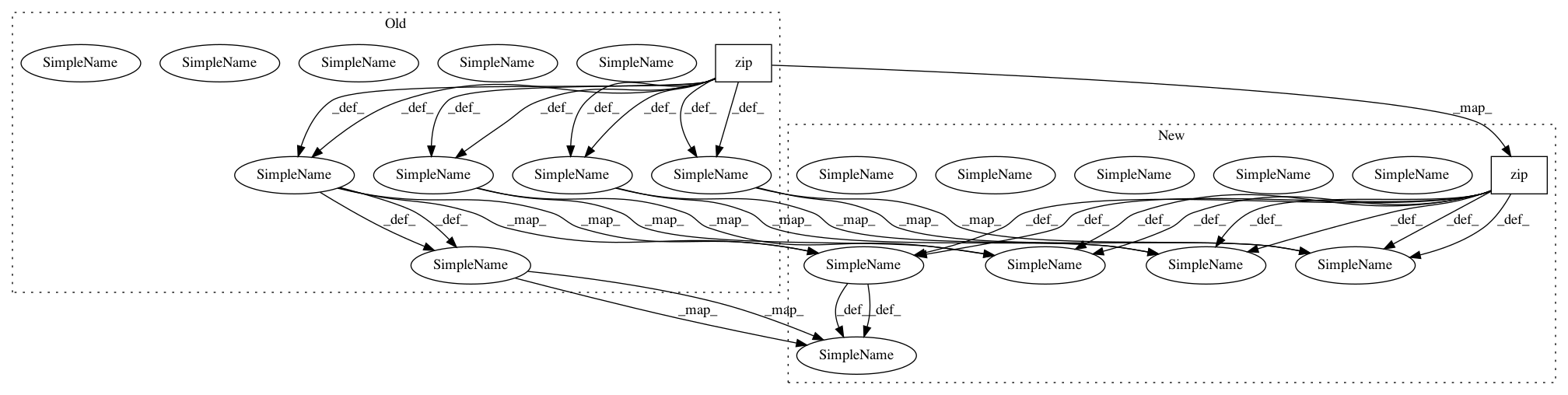
In pattern: SUPERPATTERN
Frequency: 4
Non-data size: 2
Instances
Project Name: aleju/imgaug
Commit Name: c6b8a28f10e865c8444e9e8ffb6eee5dbd428192
Time: 2019-08-25
Author: kontakt@ajung.name
File Name: test/augmenters/test_blur.py
Class Name: Test_blur_gaussian_
Method Name: test_other_dtypes_sigma_075
Project Name: aleju/imgaug
Commit Name: c6b8a28f10e865c8444e9e8ffb6eee5dbd428192
Time: 2019-08-25
Author: kontakt@ajung.name
File Name: test/augmenters/test_blur.py
Class Name: Test_blur_gaussian_
Method Name: test_other_dtypes_sigma_0
Project Name: aleju/imgaug
Commit Name: f98d7f30e941330e575412670a310a979bc21eba
Time: 2019-08-16
Author: kontakt@ajung.name
File Name: imgaug/augmenters/geometric.py
Class Name: Affine
Method Name: _augment_heatmaps
Project Name: aleju/imgaug
Commit Name: 6d27324706e7faa19c4e91255dec6c99d9c8526d
Time: 2019-08-31
Author: kontakt@ajung.name
File Name: test/augmenters/test_mixed_files.py
Class Name:
Method Name: test_keypoint_augmentation